As any other programming language you do have Python variable, values and data types and they are essential part of your python code.
Python Variable
There are some specific rules must be followed when we define Variables in python. Here are those rules to define variables –
- Variable Name must contain at least one character.
- Variable Name must contain at least one character.
- The first character can be alphabetic letter (upper case or lower case) or the underscore. e.g. AxyBSNDmkerdsladCPFAEVXZkior
- The remaining characters (if any) may be alphabetic characters (upper or lower case), the underscore, or a digit. e.g. AxyBSNDmkerdsladCPFAEVXZkiorTd_02538923
- No other characters (including spaces) are permitted.
- A reserved word cannot be used as a variable name (see below table 1.1)
- Variable names are case sensitive
Here are few examples of valid and invalid identifiers.
Valid Name : x, xY2, TekkieHead, _Myblog, _2days_BLOG, BLOG
Invalid Name :
- 5 (begins with a digit),
- @_Blog (special characters are not allowed),
- Tekkie%Head (special characters are not allowed),
- Python-Program (dash is not a legal symbol in an identifier),
- Class (reserved words are not allowed),
- Good Read (space is not a legal symbol in an identifier)
Examples:
>>> x=1000
>>> x
1000
>>> TekkieHead=”Friend of Python”
>>> TEKKIEHEAD
Traceback (most recent call last):
File “”, line 1, in
NameError: name ‘TEKKIEHEAD’ is not defined
>>> TekkieHead
‘Friend of Python’
>>>
>>> Tekkie%Head=”Special character in variale name”
File “”, line 1
SyntaxError: can’t assign to operator
>>>
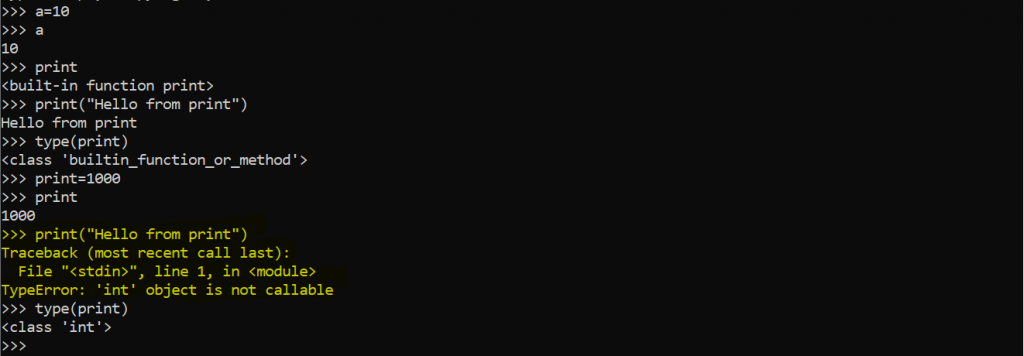
Also, there are exceptions with few words which are not special, but it happens to be Python Keywords.
You may use it as a variable name and reassign it but be careful with sequence of your code.
Python keywords : print, str, int or type
Let’s have a look what happens, if we use python keywords as a variable name.
Python Reserves 33 Keywords
and | del | from | None | True |
---|---|---|---|---|
as | elif | global | nonlocal | try |
assert | else | if | not | while |
break | except | import | or | with |
class | false | in | pass | yield |
continue | finally | is | raise | def |
for | lambda | return |
Table 1.1

Values and Data Types
A value is one of the basic things on which your program works, like a letter or a number.
The common types of values we use in our daily life as follows – Integer (Number), String (character or letter), float (numbers with decimal).
If you are not sure what exactly type of any value/variable, then you can use type () function.
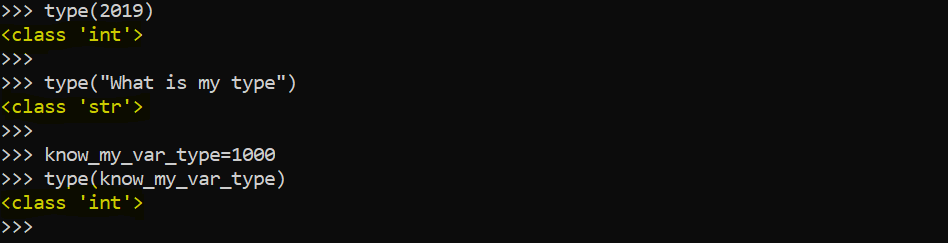
The value can be assigned to variables without defining them or declaring them at first instance.
Python take cares of everything when you assign the value. When we try to reassign the value from int to string, python changes the variable data types internally.
In below schematic diagram see how variable assignment and reassignment happens in Python.
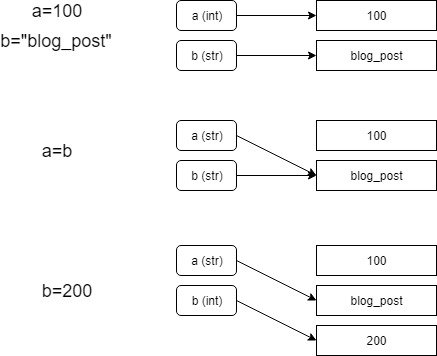